How to Run LLaMA 4 Locally
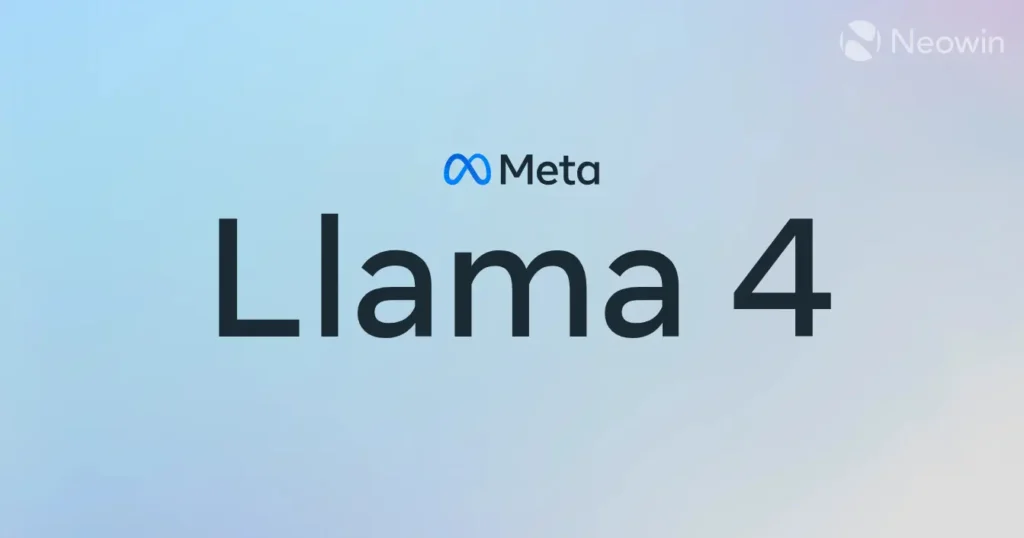
The release of Meta’s LLaMA 4 marks a significant advancement in large language models (LLMs), offering enhanced capabilities in natural language understanding and generation. For developers, researchers, and AI enthusiasts, running LLaMA 4 locally provides opportunities for customization, data privacy, and cost savings. This comprehensive guide explores the requirements, setup, and optimization strategies for deploying LLaMA 4 on your local machine.
What Is LLaMA 4?
LLaMA 4 is the latest iteration in Meta’s series of open-source LLMs, designed to deliver state-of-the-art performance in various natural language processing tasks. Building upon its predecessors, LLaMA 4 offers improved efficiency, scalability, and support for multilingual applications.
Why Run LLaMA 4 Locally?
Running LLaMA 4 on your local machine offers several advantages:
- Data Privacy: Keep sensitive information on-premises without relying on external servers.
- Customization: Fine-tune the model to suit specific applications or domains.
- Cost Efficiency: Eliminate recurring cloud service fees by utilizing existing hardware.
- Offline Access: Ensure uninterrupted access to AI capabilities without internet dependency.
System Requirements
Hardware Specifications
To run LLaMA 4 effectively, your system should meet the following minimum requirements:
- GPU: NVIDIA RTX 5090 with 48GB VRAM.
- CPU: 12-core processor (e.g., Intel i9 or AMD Ryzen 9 series).
- RAM: 64GB minimum; 128GB recommended for optimal performance.
- Storage: 2TB NVMe SSD to accommodate model weights and training data.
- Operating System: Ubuntu 24.04 LTS or Windows 11 with WSL2.
Software Dependencies
Ensure the following software components are installed:
- Python: Version 3.11.
- PyTorch: With CUDA support for GPU acceleration.
- Hugging Face Transformers: For model loading and inference.
- Accelerate: To manage training and inference processes.
- BitsAndBytes: For model quantization and memory optimization.
Setting Up the Environment
Creating a Python Environment
Begin by setting up a dedicated Python environment:
conda create -n llama4 python=3.11
conda activate llama4
Installing Required Packages
Install the necessary Python packages:
pip install torch torchvision torchaudio --extra-index-url https://download.pytorch.org/whl/cu121
pip install transformers accelerate bitsandbytes
Downloading LLaMA 4 Model Weights
To access LLaMA 4 model weights:
- Visit Meta’s official LLaMA model page.
- Request access and accept the license terms.
- Once approved, download the model weights using the provided script:
python -m huggingface_hub download meta-llama/Llama-4-8B --local-dir ./models/llama4
How to Deploy LLaMA 4 Locally
Basic Inference Setup
Implement a basic inference setup using the following Python script:
from transformers import AutoModelForCausalLM, AutoTokenizer
import torch
# Load the model and tokenizer
model_path = "./models/llama4"
tokenizer = AutoTokenizer.from_pretrained(model_path)
model = AutoModelForCausalLM.from_pretrained(
model_path,
torch_dtype=torch.float16,
device_map="auto"
)
# Define an inference function
def generate_text(prompt, max_length=512):
inputs = tokenizer(prompt, return_tensors="pt").to("cuda")
outputs = model.generate(
**inputs,
max_length=max_length,
temperature=0.7,
top_p=0.9,
do_sample=True
)
return tokenizer.decode(outputs[0], skip_special_tokens=True)
# Example usage
test_prompt = "Explain the concept of artificial intelligence:"
print(generate_text(test_prompt))
Optimizing for RTX 5090
Leverage the capabilities of the RTX 5090 GPU by enabling flash attention and 8-bit quantization:
# Enable flash attention
model.config.attn_implementation = "flash_attention_2"
# Apply 8-bit quantization
from transformers import BitsAndBytesConfig
quantization_config = BitsAndBytesConfig(
load_in_8bit=True,
llm_int8_threshold=6.0
)
model = AutoModelForCausalLM.from_pretrained(
model_path,
quantization_config=quantization_config,
device_map="auto"
)
Fine-Tuning LLaMA 4
Preparing Training Data
Structure your training data in JSONL format:
import json
# Sample dataset
dataset = [
{
"instruction": "Define machine learning.",
"input": "",
"output": "Machine learning is a subset of artificial intelligence that focuses on..."
},
# Add more entries as needed
]
# Save to a JSONL file
with open("training_data.jsonl", "w") as f:
for entry in dataset:
f.write(json.dumps(entry) + "\n")
Implementing Parameter-Efficient Fine-Tuning (PEFT)
Utilize PEFT with LoRA for efficient fine-tuning:
from peft import prepare_model_for_kbit_training, LoraConfig, get_peft_model
from transformers import TrainingArguments, Trainer
# Prepare the model
model = prepare_model_for_kbit_training(model)
# Configure LoRA
lora_config = LoraConfig(
r=16,
lora_alpha=32,
target_modules=["q_proj", "k_proj", "v_proj", "o_proj"],
lora_dropout=0.05,
bias="none",
task_type="CAUSAL_LM"
)
# Apply LoRA
model = get_peft_model(model, lora_config)
# Define training arguments
training_args = TrainingArguments(
output_dir="./results",
num_train_epochs=3,
per_device_train_batch_size=4,
gradient_accumulation_steps=4,
learning_rate=2e-4,
weight_decay=0.01,
warmup_steps=100,
save_steps=500,
logging_steps=50,
fp16=True
)
# Initialize the Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=dataset,
data_collator=data_collator
)
# Start training
trainer.train()
Monitoring Training Progress
Install and launch TensorBoard to monitor training:
pip install tensorboard
tensorboard --logdir=./results/runs
Access TensorBoard at http://localhost:6006/
.
Evaluating the Fine-Tuned Model
After fine-tuning, evaluate the model’s performance:
from peft import PeftModel
# Load the base model
base_model = AutoModelForCausalLM.from_pretrained(
model_path,
torch_dtype=torch.float16,
device_map="auto"
)
# Load the fine-tuned model
fine_tuned_model = PeftModel.from_pretrained(
base_model,
"./results/checkpoint-1000"
)
# Merge weights
merged_model = fine_tuned_model.merge_and_unload()
# Evaluate on test prompts
test_prompts = [
"Explain reinforcement learning.",
"Discuss ethical considerations in AI."
]
for prompt in test_prompts:
inputs = tokenizer(prompt, return_tensors="pt").to("cuda")
outputs = merged_model.generate(
**inputs,
max_length=512,
temperature=0.7,
top_p=0.9,
do_sample=True
)
print(f"Prompt: {prompt}")
print(f"Response: {tokenizer.decode(outputs[0], skip_special_tokens=True)}")
print("-" * 50)
Performance Optimization Strategies
Memory Management
Implement gradient checkpointing and mixed precision training to optimize memory usage:
# Enable gradient checkpointing
model.gradient_checkpointing_enable()
# Configure training arguments
training_args = TrainingArguments(
fp16=True,
bf16=False,
optim="adamw_torch",
# Additional arguments...
)
Troubleshooting Common Issues
CUDA Out of Memory Errors:
- Reduce batch size.
- Enable gradient checkpointing.
- Utilize 8-bit quantization.
- Implement gradient accumulation.
Slow Training Performance:
- Enable flash attention.
- Increase batch size if memory permits.
- Offload operations to the CPU.
- Integrate DeepSpeed for multi-GPU setups.
Conclusion
Deploying and fine-tuning LLaMA 4 locally empowers you with a robust AI tool tailored to your specific needs. By following this guide, you can harness the full potential of LLaMA 4, ensuring data privacy, customization, and cost-effective AI solutions.
Getting Started
CometAPI provides access to over 500 AI models, including open-source and specialized multimodal models for chat, images, code, and more. Its primary strength lies in simplifying the traditionally complex process of AI integration.
CometAPI offer a price far lower than the official price to help you integrate Llama 4 API, and you will get $1 in your account after registering and logging in! Welcome to register and experience CometAPI.CometAPI pays as you go,Llama 4 API in CometAPI Pricing is structured as follows:
Category | llama-4-maverick | llama-4-scout |
API Pricing | Input Tokens: $0.48 / M tokens | Input Tokens: $0.216 / M tokens |
Output Tokens: $1.44/ M tokens | Output Tokens: $1.152/ M tokens |
- Please refer to Llama 4 API for integration details.
Start building on CometAPI today – sign up here for free access or scale without rate limits by upgrading to a CometAPI paid plan.